728x90
딥러닝은 가장 적합한 가중치를 찾는과정
사용용도에따라 다양한 모델이 있다 (CNN, DNN, RNN)
RNN은 주로 시계열 데이터에 사용된다. (시간의 흐름에 따라 이전 결과에 영향을 계속 받음)
CNN은 주로 이미지를 분석할때 사용 (차원감소)
신경망의 종류
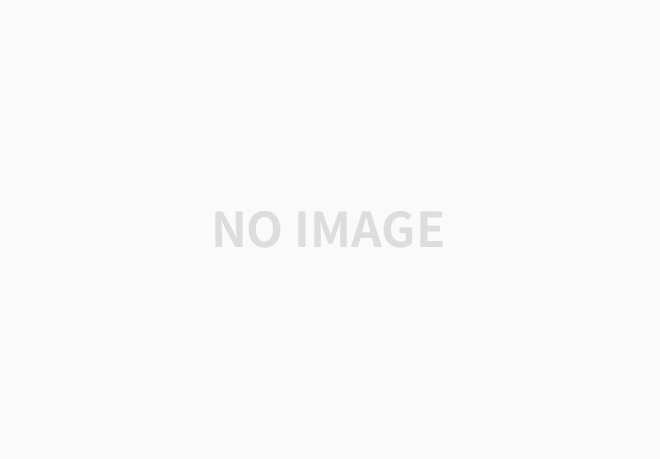
⦿ 시계열 데이터에 주로 사용됨. 앞의 결과가 뒤의 결과에 영향을 줌
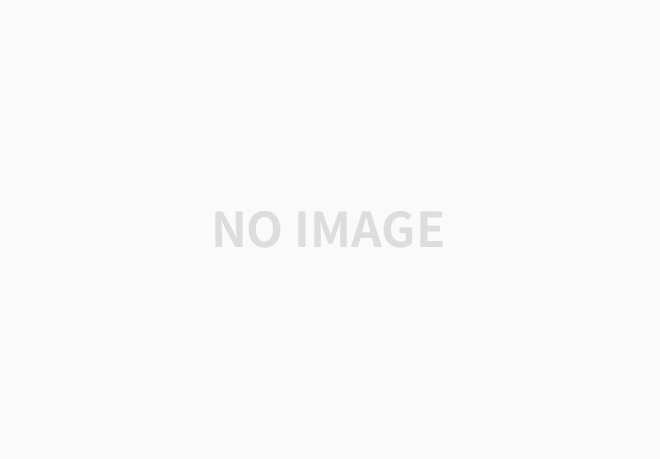
⦿ 이미지 분석할때 항상 나옴
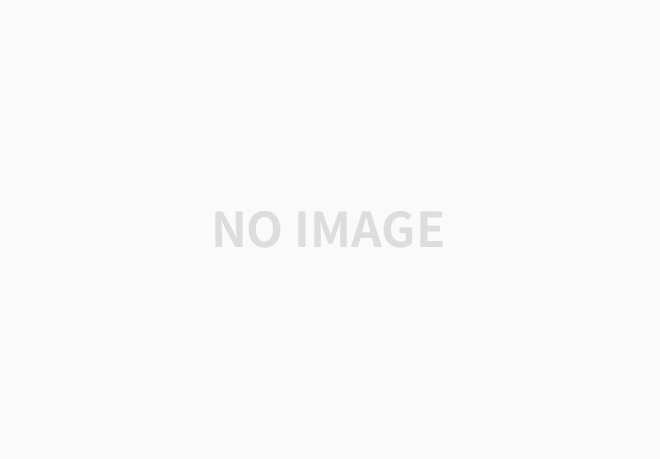
신경망 기초
함수로 표현하기
# 신경망 기초
import math
import numpy as np
import matplotlib.pyplot as plt
# 일차함수
def linear_function(x):
a=0.5
b=2
return a*x+b
x = np.arange(-5,5,0.1)
y = linear_function(x)
plt.plot(x,y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Linear Function')
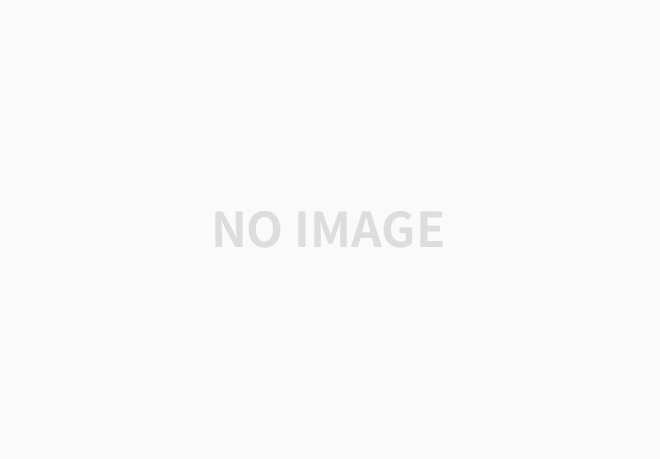
# 2차 함수
def quadratic_fuction(x):
a = 1
b = -1
c = -2
return a*x**2 + b*x + c
x = np.arange(-5,5,0.1)
y = quadratic_fuction(x)
plt.plot(x,y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Quadratic Function')

# 3차 함수
def cubic_function(x):
a = 4
b = 0
c = -1
d = -8
return a*x**3 + b*x**2 + c*x + d
x = np.arange(-5,5,0.1)
y = cubic_function(x)
plt.plot(x,y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Cublic Function')
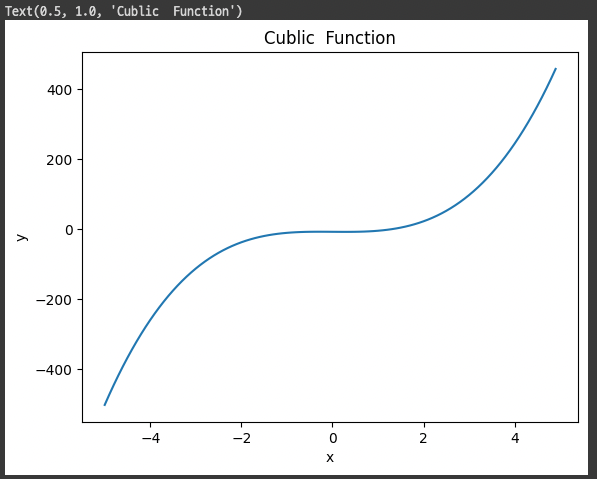
텐서의 표현
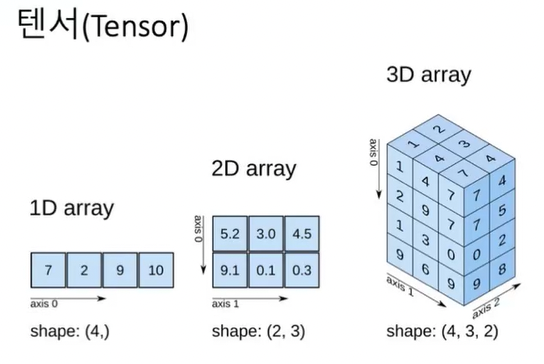
# Tensor의 표현 (스칼라와 벡터)
# Tensor의 표현 # 스칼라와 벡터
x = np.array(3) #스칼라
print(x)
print(x.shape) # 형태, 모양
print(x.ndim) # 차원
# 3
# ()
# 0
y = np.array([3]) # 벡터
print(y)
print(y.shape)
print(y.ndim)
# [3]
# (1,)
# 1
# 1차원 텐서
a = np.array([1,2,3,4])
b = np.array([5,6,7,8])
c = a + b
d = d + x # x = 3 d(1차원과) + x(0차원) 합도 가능
print(c)
print(c.shape)
print(c.ndim)
# [ 6 8 10 12]
# (4,)
# 1
print(d)
print(d.shape)
print(d.ndim)
# [4 5 6 7]
# (4,)
# 1
# 차원이 달라서 부족한 값들은 그냥 같은값을 복사해서 연산한다. 브로드캐스팅이라 한다.
# 똑같이 하나의 성분이라도 리스트에 포함시키면 1차원이 되므로 벡터가 된다.
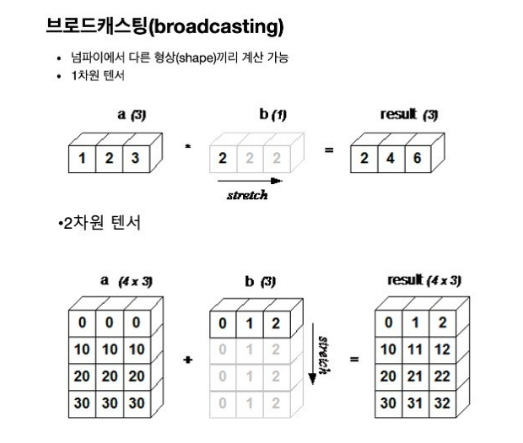
# 벡터의 곱
# 벡터의 곱
x = np.array([1,2,0])
y = np.array([0,2,1])
z = np.dot(x, y)
print(z)
print(z.shape)
print(np.ndim(z))
# 4
# ()
# 0
# 전치 행렬
# 전치 행렬
a = np.array([[1,2,3],[4,5,6]])
print(a)
print(a.shape)
# [[1 2 3]
[4 5 6]]
# (2, 3)
a_ = a.T # 전치행렬로 행과 열을 바꿈
print(a_)
print(a_.shape)
# [[1 4]
[2 5]
[3 6]]
# (3, 2)
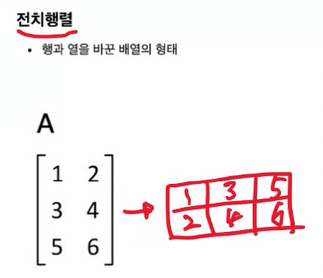
# 2차원 텐서
# 2차원 텐서
matrix = np.array([[1,2,3],[4,5,6]])
print(matrix)
print(matrix.shape)
print(np.ndim(matrix))
# [[1 2 3]
[4 5 6]]
# (2, 3)
# 2
a = np.array([[1,2],[3,4]])
b = np.array([[10,10],[10,10]])
print(a * b)
# [[10 20]
[30 40]]
# 3차원 텐서
# 3차원 텐서
x = np.array([[[5,3,2,1],
[5,5,3,1],
[6,1,2,3]],
[[1,1,1,1],
[3,4,7,5],
[1,8,3,4]],
[[10,9,3,9],
[5,4,3,2],
[7,6,3,4]]])
print(x)
print(x.shape)
print(np.ndim(x))
#[[[ 5 3 2 1]
[ 5 5 3 1]
[ 6 1 2 3]]
[[ 1 1 1 1]
[ 3 4 7 5]
[ 1 8 3 4]]
[[10 9 3 9]
[ 5 4 3 2]
[ 7 6 3 4]]]
# (3, 3, 4)
# 3

@ 이미지는 가로축, 세로축, 색상값 의 3차원 데이터이다.
@ [w, h, [r,g,b]] 의 형태이다.
@ 시계열 데이터도 3차원텐서를 사용한다. 시계열데이터란 한쪽축이 시간축으로 시간에따른 데이터들의 변화를 나타내는 것을 의미한다. 주식, 기상 등의 값이있다.
@ 영상은 4차원텐서 w, h, f(프레임), [r,g,b] 의 형태
[4차원, 5차원 텐서]
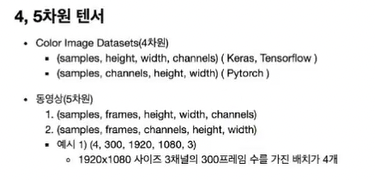
신경망의 구조

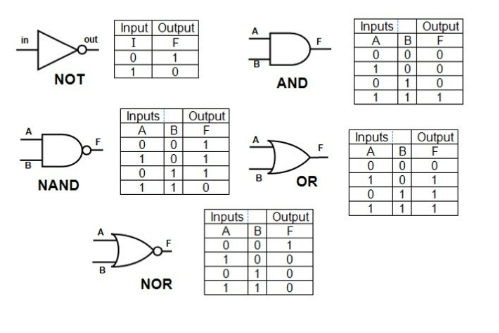
# 논리게이트
# AND 게이트
# 논리 게이트
# AND
def AND(a,b):
input = np.array([a,b])
weights = np.array([0.4, 0.4]) # 가중치 +
bias = -0.6 # 편향 강한 -
value = np.sum(input * weights) + bias
if value <= 0:
return 0
else:
return 1
print(AND(0,0))
print(AND(0,1))
print(AND(1,0))
print(AND(1,1))
# 0
# 0
# 0
# 1
가중치와 바이어스를 컴퓨터가 스스로 찾도록 하는것이 딥러닝
x1 = np.arange(-2,2,0.01)
x2 = np.arange(-2,2,0.01)
bias = -0.6
y = (-0.4 * x1 - bias) / 0.4
plt.axvline(x=0)
plt.axhline(y=0)
plt.plot(x1, y, 'r--')
plt.scatter(0,0,color='orange', marker='o',s=150)
plt.scatter(0,1,color='orange', marker='o',s=150)
plt.scatter(1,0,color='orange', marker='o',s=150)
plt.scatter(1,1,color='black', marker='^',s=150)
plt.xlim(-0.5,1.5)
plt.ylim(-0.5,1.5)
plt.grid()
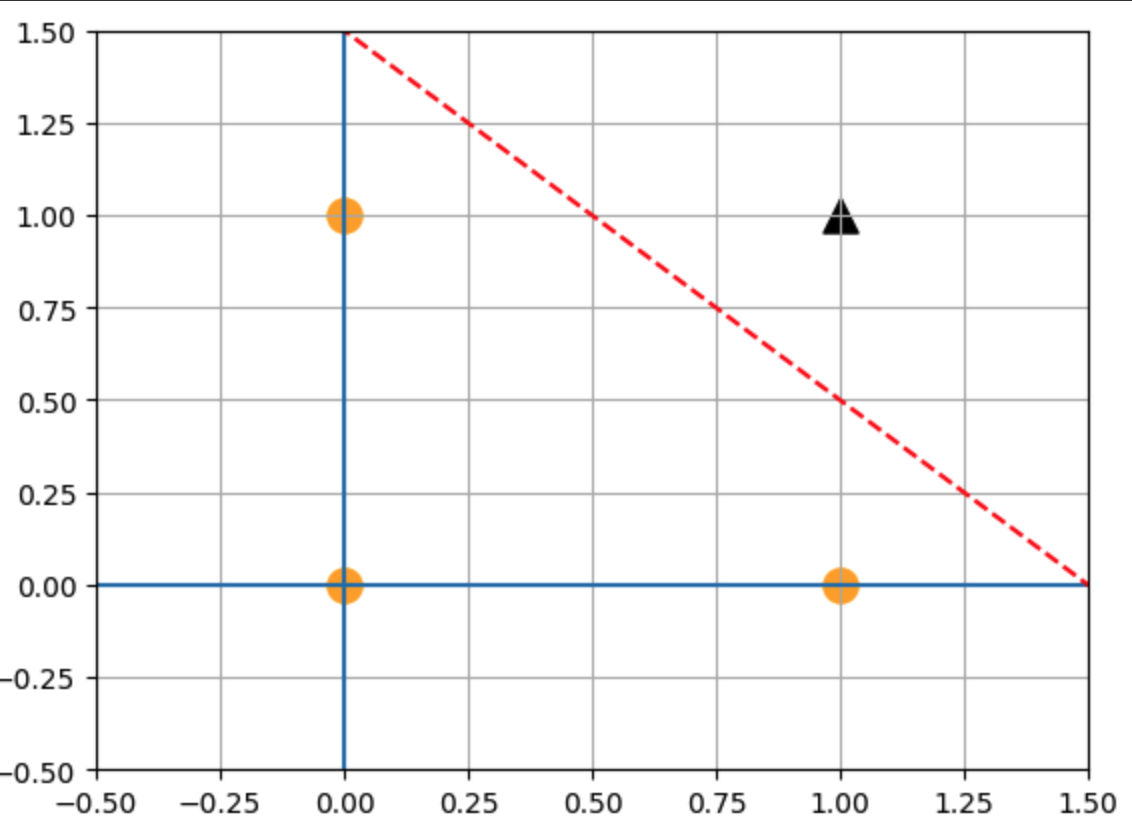
# OR 게이트
# OR
def OR(a,b):
input = np.array([a,b])
weights = np.array([0.4, 0.4]) # 가중치 +
bias = -0.3 # 편향 약한 -
value = np.sum(input * weights) + bias
if value <= 0:
return 0
else:
return 1
print(OR(0,0))
print(OR(0,1))
print(OR(1,0))
print(OR(1,1))
# 0
# 1
# 1
# 1
# NAND 게이트
# NAND
def NAND(a,b):
input = np.array([a,b])
weights = np.array([-0.5, -0.5]) # 가중치 -
bias = 0.7 # 편향은 강한 +
value = np.sum(input * weights) + bias
if value <= 0:
return 0
else:
return 1
print(NAND(0,0))
print(NAND(0,1))
print(NAND(1,0))
print(NAND(1,1))
# 1
# 1
# 1
# 0
x1 = np.arange(-2, 2, 0.01)
x2 = np.arange(-2, 2, 0.01)
bias = 0.7
y = (0.6 * x1 - bias) / -0.5
plt.axvline(x=0)
plt.axhline(y=0)
plt.plot(x1, y, 'r--')
plt.scatter(0,0,color='black',marker='o',s=150)
plt.scatter(0,1,color='black',marker='o',s=150)
plt.scatter(1,0,color='black',marker='o',s=150)
plt.scatter(1,1,color='orange',marker='^',s=150)
plt.xlim(-0.5,1.5)
plt.ylim(-0.5,1.5)
plt.grid()
plt.show()
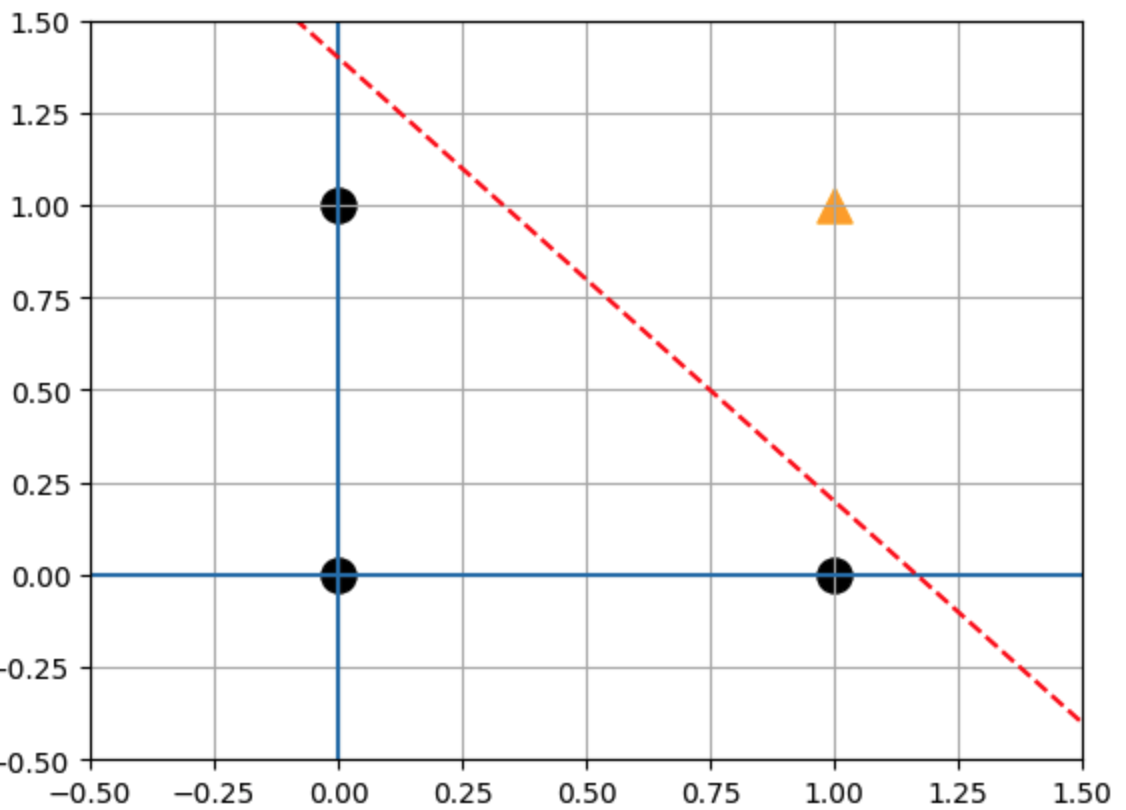
# XOR 게이트
def XOR(x1, x2):
s1 = NAND(x1, x2)
s2 = OR(x1, x2)
y = AND(s1, s2)
return y
print(XOR(0,0))
print(XOR(0,1))
print(XOR(1,0))
print(XOR(1,1))
# 0
# 1
# 1
# 0
728x90