728x90
1. 이미지 타입, 크기 확인하기
import cv2
image_path = "./data/cat.png"
# image read
image = cv2.imread(image_path)
# image 타입
image_type = type(image)
print("이미지 타입 >> " , image_type)
# 이미지 크기 확인
image_height, image_width, image_channel = image.shape
print(image_height, image_width, image_channel)
# 이미지 타입 >> <class 'numpy.ndarray'>
# 162 310 3
2. 이미지 리사이징
import cv2
import matplotlib.pyplot as plt
image_path = "./data/cat.png"
image = cv2.imread(image_path)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB) # cv2로 불러오면 BGR을 RGB로 바꿔줘야함
# https://itcreator.tistory.com/141 에 적어둠
# 이미지 크기 조절
image_resize = cv2.resize(image, (244,244))
# 이미지 크기 확인
image_height_, image_width_, _ = image_resize.shape
# 원본 이미지
plt.imshow(image)
plt.show()
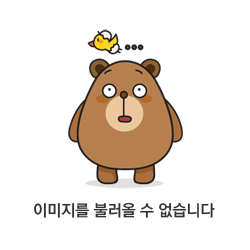
# 리사이즈 이미지
print(image_height_, image_width_)
# 244 244
plt.imshow(image_resize)
plt.show()
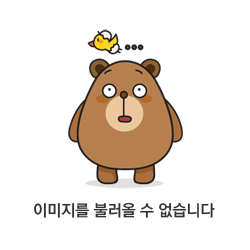
# 저장하기
import cv2
image_resize = cv2.cvtColor(image_resize, cv2.COLOR_BGR2RGB)
cv2.imwrite("./data/resize_image.png", image_resize) # cv2.imwrite로 저장하기
# True라고 뜨면 저장된거
3. 이미지 자르기(슬라이싱)
import cv2
import matplotlib.pyplot as plt
image_path = "./data/cat.png"
image = cv2.imread(image_path)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image_cropped = image[10:,:200] # [행시작:행끝, 열시작:열끝]
plt.imshow(image)
plt.show()
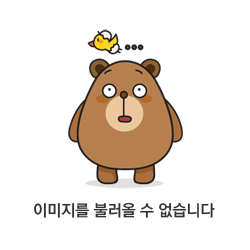
plt.imshow(image_cropped)
plt.show()
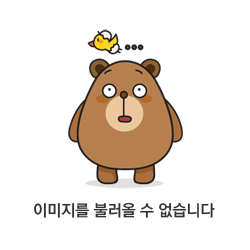
3-1. 이미지자르기(좌표)
import cv2
import matplotlib.pyplot as plt
image_path = "./data/cat.png"
image = cv2.imread(image_path)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
x1, y1 = 50, 50 # 좌측 상단 모서리 좌표
x2, y2 = 150,150 # 우측 하단 모서리 좌표
cropped_image_test = image[y1:y2, x1:x2] # y가 먼저인 이유는 좌표평면에서 행렬의 행은 y값임
plt.imshow(cropped_image_test)
plt.show()
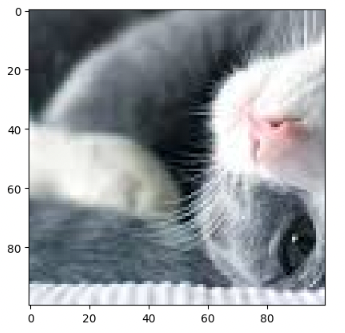
2. 변환하기
3. 리사이즈
4. 크롭 (슬라이싱,좌표)
728x90
'ComputerVision > [CV]' 카테고리의 다른 글
[CV] 이미지 기본세팅 4 (이미지 회전, 상하좌우반전) (1) | 2023.06.08 |
---|---|
[CV] 이미지 기본세팅 3 (블러, 선명도, 대비) (2) | 2023.06.08 |
[CV] 이미지 기본세팅 1 (불러오기, 저장하기) (0) | 2023.06.07 |
[CV] SAM - Segment Anything Model by META (0) | 2023.04.06 |
[CV] Pytorch VS tensorflow(keras) 점유율 비교 (0) | 2023.04.03 |