728x90
DataFrame에서 데이터 추출
1. 추출용 데이터 셋 다운받기
https://www.kaggle.com/competitions/titanic/data
에서 자료 다운받기
# 데이터셋 불러오기
import pandas as pd
titan_df = pd.read_csv('./titanic/train.csv')
titan_df
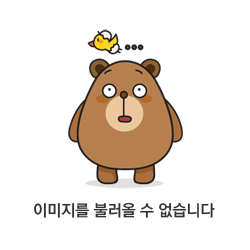
2. 선택된 열(column) 세로만 뽑아보기
# 선택된 열(column) 세로만 뽑아보기
titan_df[['Name']]
# 여러개는 ,'컬럼명' 넣어주면 된다
# titan_df[['Name','Age']]
# print(type(titan_df[['Name']])) => [[]] 2차원 <class 'pandas.core.frame.DataFrame'>
# print(type(titan_df['Name'])) => [] 1차원 <class 'pandas.core.series.Series'>
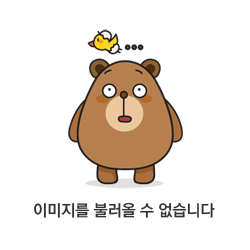
2-1. 해당조건의 열(column) 세로 뽑아보기
# 3. Pclass 1인 정보 추출하기
titan_df[titan_df.Pclass==1] # <class 'pandas.core.series.Series'>
# titan_df[titan_df['Pclass']==1] # 이렇게 해도됨
#titan_df[titan_df.Pclass==1].Pclass.value_counts() # 갯수
# print(type(titan_df[titan_df.Pclass==1])) => <class 'pandas.core.frame.DataFrame'>
# print(type(titan_df.Pclass==1])) => <class 'pandas.core.series.Series'>
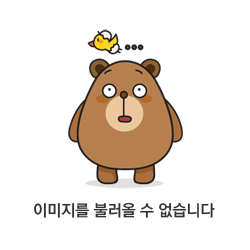
여러가지 조건
# and 조건일 때는 & / or 조건일 때는 | / Not 조건일 때는 ~
# 60세넘는 1등급 여성 추출
titan_df[(titan_df.Pclass==1) & (titan_df.Sex=='female') & (titan_df['Age'] > 60)]
# Not 조건인 경우 (반대조건)
# titan_df[~((titan_df.Pclass==1) & (titan_df.Sex=='female') & (titan_df['Age'] > 60))]
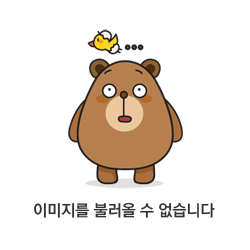
2-2 여러가지 기능들
# 해당 열(Name)에 글자가 포함된 데이터 출력
titan_df[titan_df.Name.str.contains('Mrs')]
# 인덱스번호 재정렬
titan_df[titan_df.Name.str.contains('Mrs')].reset_index()
# 인덱스번호 재정렬상태에서 상위3개만 보여주기
# titan_df[titan_df.Name.str.contains('Mrs')].reset_index().head(3) # 상위 3개
# titan_df[titan_df.Name.str.contains('Mrs')].reset_index()[:3] # 상위 3개
# titan_df[titan_df.Name.str.contains('Mrs')].reset_index().tail(3) # 하위
# 결측치받기
.isna() # 표로 보여주기
.isna().sum() # 합쳐서 보여주기
3. 선택된 행(row) 가로만 뽑아보기
titan_df.loc[[0]] # loc 이름이 0인 행만 추출해줘
titan_df.iloc[[0]] # iloc 위치가 0번째 행인 값들만 추출해줘
# 단일값을 불러오기1 - loc(label::명칭) // iloc(Positional-위치)
titan_df.loc[[0]] # loc 이름이 0인 행만 추출해줘
titan_df.iloc[[0]] # iloc 위치가 0번째 행인 값들만 추출해줘
# iloc 경우 슬라이스 가능
# iloc [시작:끝:옵션]
# 테스트하기 위해서 행 이름 바꿀때
# data_df.rename(index={'two': 1, 'one': 3}, inplace=True) # inplace 원본도 바꿀래?
data_df

3-1. loc/iloc 을 통한 행열 을 사용하여 하나의 값 뽑기
titan_df.loc[0,'Pclass']
# 3 출력
# titan_df.loc[[0,'Pclass']] 로 하면 "['Pclass'] not in index" 에러 뜸
3-2. loc/iloc 을 통한 행열 을 사용하여 여러개의 값 뽑기
titan_df.loc[:,'Pclass':'Age']
# 행 [:] = 전부 , 열 ['Pclass':'Age'] Pclass부터 ~ Age 까지3-1. loc을 통한 행열 을 사용하여 하나의 값 뽑기
titan_df.iloc[:,2:6]
# 와 동일한 값 (2열부터 ~ 2,3,4,5 까지) 6열 포함x
<원본>
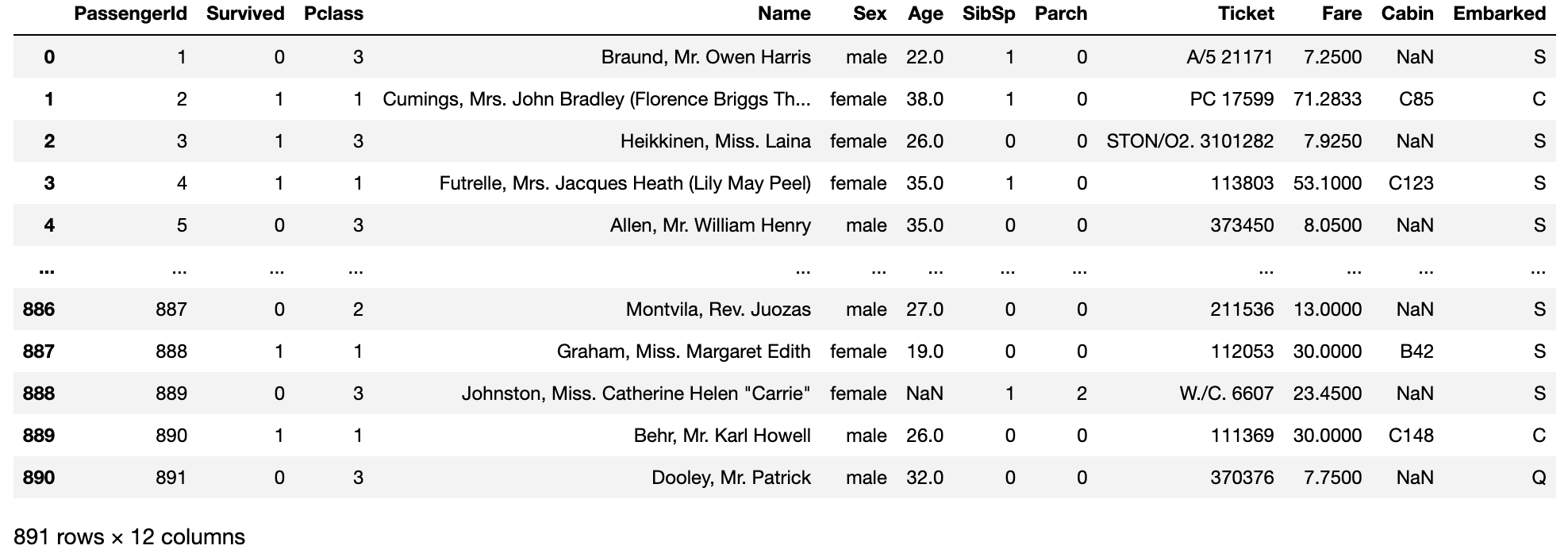
<조건으로 나온 값>
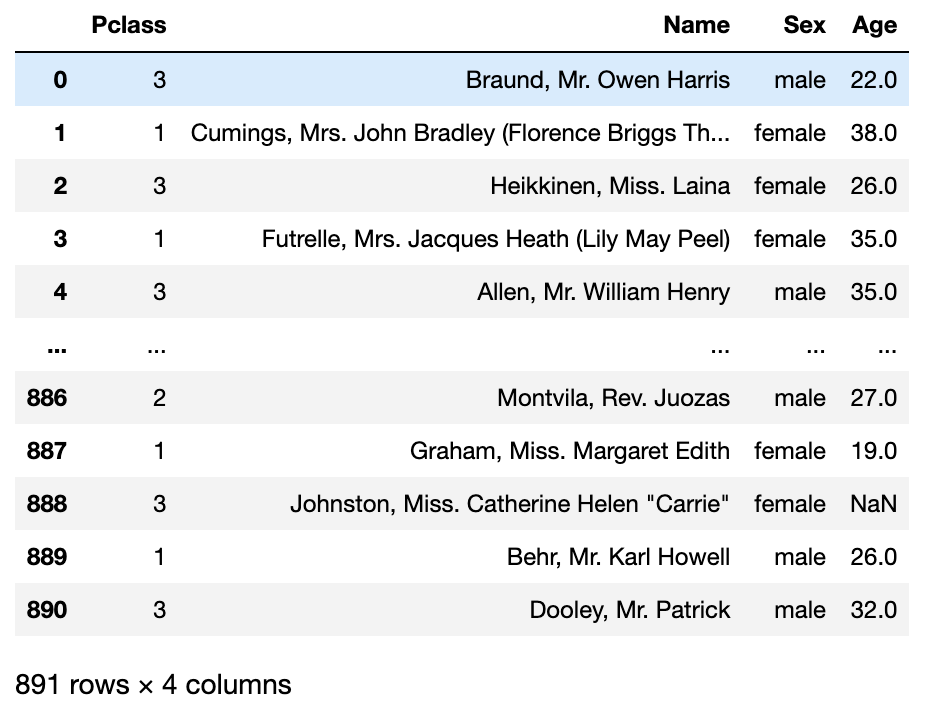
728x90
'AI > [Pandas]' 카테고리의 다른 글
[Pandas] 5. 데이터 값 변경 및 함수 + 문제 (U) (0) | 2023.02.03 |
---|---|
[Pandas] 3. DataFrame 행(row)열(column) 추가 삭제 (C, D) (0) | 2023.02.03 |
[Pandas] 2. Pandas 시리즈(Series)와 데이터프레임(Dataframe) (2) | 2023.02.03 |
[Pandas] 1. 행렬 이거 하나로 한방정리(가로?세로?) (0) | 2023.02.03 |